Beginner Java Programming - Episode 3: Tic Tac Toe. We utilize all of the tricks we learned in the Java tutorial on Arrays & For Loops and cover Methods and return types. Tic Tac Toe In.
- Hi I am writing a tic tac toe game. I have spelled out in comments in my code what I need. What I am having trouble with right now is making a getMove method. I assume I would need to call the getM.
- A Beginner Tic-Tac-Toe Class for Java Posted on 04/27/14 Desktop Programming, General Discussion, Programming Theory Around this time each year we mentors on Dream.In.Code see a bunch of students and beginners, attempting to do there final programming projects, come on the boards asking for help with coding problems.
The program I have below is a Tic Tac Toe game. Here are the details of the assignment:
TicTacToe
- Store the game board in a single 2D array. (3 x 3) X
- method to add a move.
- method to display the board.
- method to tell whose turn it is (X or O).
- method to find a winner or tie.
- method to initialize the game to the beginning.
- Main method that allows two players to enter their turns on the same keyboard.
My problem is that I don't know how to take coordinates entered by the user and turn them into an 'X' or 'O' value for the user's turn into the array which it then displays onto the board itself for the user after they make each play. There are compiling errors on line; ticTac.showBoard(char[][] displayArray);. Any other comments and errors you have on how to simplify things or errors you see are definitely welcome!
2 Answers
I don't know how to take the coordinates entered by the user and turn it into an x or o value for the play into the array and then displaying the board itself.
You are most of the way there in readInput
. You have the row
and col
coordinates input from the user. In the same way you look at the value in board[row][col]
when displaying the board, and board[row][0]
when checking for a win, you want to set the location in board
array to the current player. This can be done by saying board[something][something] = value
. What should something
and value
be?
Any other comments and errors you have on how to simplify things or errors you see are definitely welcome!
It looks like almost all of the pieces of the program are there and completed. However, the flow of the program tying everything together has a few problems. There are a couple of principles of programming that may apply here:
- SRP (Single Responsibility Principle): Each unit of a program should have only a single purpose
- SoC (Separation of Concerns): Each module or unit should have a clear dividing line. Two methods should not partially overlap in what they do.
With these ideas in mind, I will offer a couple of comments on your program flow:
- Often you can tell if you have a clean design if the names of the methods are what they actually do. For example,
findResult()
makes a call tosetPlayerTurn()
. That is misleading because the current player changes every time you simply want to check the game result. When exactly should you switch turns? - Who's job is it to display the board? You are displaying the board in the main program, but you never see that because
readInput()
loops 8 times before you ever reach the call toshowBoard()
. Showing the board should probably be the responsibility ofTicTacToe
. Themain
method should just kick off the game. - Does
readInput()
read input, or play the whole game? It sounds like it should read one set of coordinates input, but it has a loop that processes the whole game. It may make more sense to separate that into two methods - one that handles a single move, and one that loops through 9 times, displaying the board and calling thereadInput()
method.
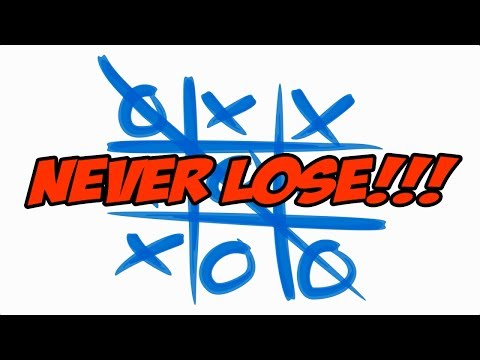
Change your call to showboard in your main to
Since you already have the board within a TicTacToe object.
Then showBoard() should look like this:
The reason for this is because your TicTacToe object already has a board, you do not need to pass it one.
Also, as another tip, you don't need another class to run your TicTacToe. You can simply put the main inside of TicTacToe, like so:
It's a little confusing at first to be creating an instance of your class in the main of your class but you'll get used to it...and you'll have a lot less files :-).
A note of caution when doing this though, you do need to create an object of your class to use its methods within your main because main is a 'static' method. static methods are allocated before everything else in the program and main is the first that runs in a java program so, if you don't create the object, main doesn't know how to get access to the methods inside of it. Hopefully that didn't cause more confusion than it was worth.
As another pointer, if you really were trying to create a new 2d char array to pass to that method you could have done it like so
Hypothetical inthe ClavicleHypothetical inthe ClavicleTic Tac Toe Code In Java
Not the answer you're looking for? Browse other questions tagged java or ask your own question.
Create A Tic Tac Toe Game Java
I've run into some trouble while trying to code a simple tic-tac-toe program. My issue lies in the checkForWinner method, which was provided to us as part of the exercise. When I try to compile the program gives me the following error message:
The following is the code:
The error tells me that I should be using type char, but when I've tried changing the arrays to be of type char it tells me that the following bits have incompatible types:
And:
How can I change the code to be compatible with char data and why am I getting these errors?
*I'm not trying to change the checkForWinner method to accept String data as it was provided and no changes can be made to it. *
3 Answers

The problem is - board[i][2] != ' '
. It should be board[i][2] != ' '
instead.
' '
is a char
while board[i][2]
is a String
.
Also, board[i][0] board[i][1]
is bug, do board[i][0].equals(board[i][1])
instead. And for the not-equals do !board[i][2].equals(' ')
.
The ' ' is interpreted as a string literal. If you want char values, use the single quotes ' '
I see two problems here (and in similar lines):
Here board[i][2] != ' '
you are comparing a String (double quotes) with a char (single quotes). Use double quotes for String literals.
Also, when comparing Strings, use .equals()
. The operator will only return true if the two Strings are the same object, i.e. they share the same space in memory.
So, that if statement should look like: